弹窗输入密码的实现方法
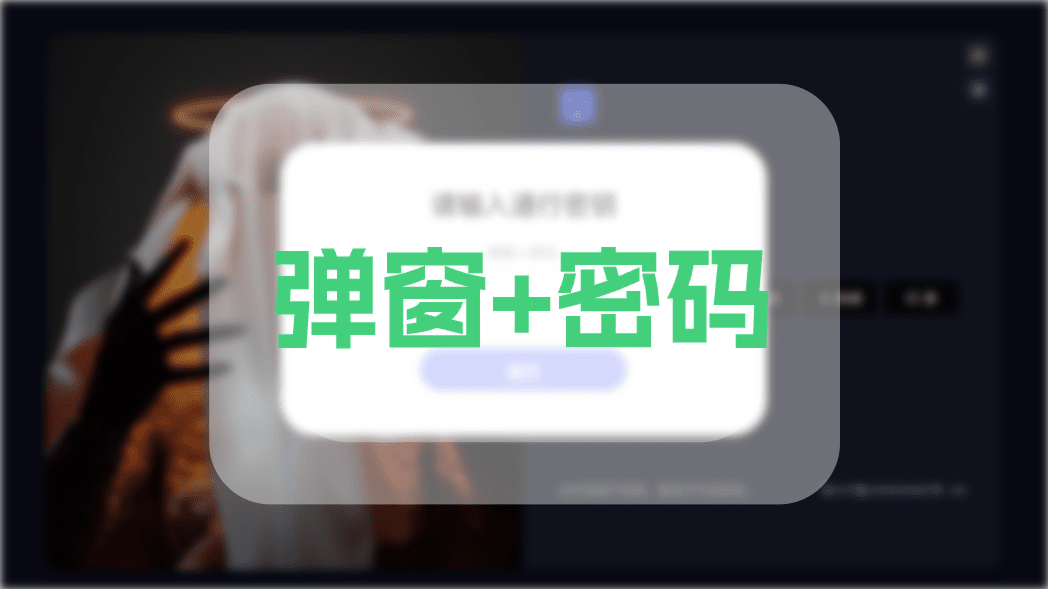
弹窗输入密码的实现方法
YuanMo预览
实现代码
1 | <div id="password-modal" style="display: none;position:fixed;top:0;left:0;width:100%;height:100%;background:rgba(0,0,0,.3);z-index:99999"> |
当然光有这个还不行,还得有js1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101const MI = "123";
// 密码设置,在“”内设置密码
window.onload = function() {
var modal = document.getElementById("password-modal");
var submitButton = document.getElementById("submit-button");
var passwordInput = document.getElementById("password-input");
var passwordAttempts = 0;
var lockoutExpiration;
// 获取存储在本地的密码状态和过期时间戳
var passwordStatus = localStorage.getItem("passwordStatus");
var expirationTimestamp = localStorage.getItem("expirationTimestamp");
var currentTime = new Date().getTime();
if (passwordStatus === "locked") {
// 检查是否到达锁定期限
if (currentTime < expirationTimestamp) {
// 如果在锁定期内,禁用密码输入框和提交按钮
passwordInput.disabled = true;
submitButton.disabled = true;
// 显示倒计时
var secondsRemaining = Math.round((expirationTimestamp - currentTime) / 1000);
var countdownInterval = setInterval(function() {
secondsRemaining--;
if (secondsRemaining === 0) {
// 解除锁定
passwordInput.disabled = false;
submitButton.disabled = false;
localStorage.removeItem("passwordStatus");
clearInterval(countdownInterval);
}
var minutes = Math.floor(secondsRemaining / 60);
var seconds = secondsRemaining % 60;
alert("密码错误三次. 请 " + minutes + " 分 " + seconds + " 秒后重新尝试输入");
},
1000);
} else {
// 如果已经超过锁定期,解除锁定
localStorage.removeItem("passwordStatus");
localStorage.removeItem("expirationTimestamp");
}
} else if (expirationTimestamp && currentTime < expirationTimestamp) {
// 如果存在过期时间戳,且当前时间小于它,直接显示网页内容
modal.style.display = "none";
document.body.style.display = "block";
} else {
// 显示密码输入框
modal.style.display = "block";
}
// 当点击“提交”按钮时,检查输入的密码是否正确
submitButton.onclick = function() {
if (passwordInput.value === MI) {
// 如果密码正确,隐藏密码输入框,显示网页内容
modal.style.display = "none";
document.body.style.display = "block";
// 重置密码状态和过期时间戳
passwordAttempts = 0;
localStorage.removeItem("passwordStatus");
localStorage.setItem("expirationTimestamp", new Date().getTime() + (120 * 60 * 60 * 1000)); // 120小时后过期
} else {
// 如果密码错误,提示用户重新输入
passwordAttempts++;
if (passwordAttempts >= 3) {
// 锁定密码状态
passwordInput.disabled = true;
submitButton.disabled = true;
lockoutExpiration = new Date().getTime() + 600000; // 10分钟后
localStorage.setItem("passwordStatus", "locked");
localStorage.setItem("expirationTimestamp", lockoutExpiration);
// 显示倒计时
var countdownInterval = setInterval(function() {
var currentTime = new Date().getTime();
var secondsRemaining = Math.round((lockoutExpiration - currentTime) / 1000);
if (secondsRemaining <= 0) {
// 解除锁定
passwordInput.disabled = false;
submitButton.disabled = false;
localStorage.removeItem("passwordStatus");
clearInterval(countdownInterval);
}
var minutes = Math.floor(secondsRemaining / 60);
var seconds = secondsRemaining % 60;
alert("密码错误三次. 请 " + minutes + " 分 " + seconds + " 秒后重新尝试输入");
},
1000);
} else {
alert("密码不正确,请重试。");
passwordInput.value = "";
}
}
};
// 防止用户直接按 Enter 键提交密码
passwordInput.onkeydown = function(event) {
if (event.keyCode === 13) {
event.preventDefault();
submitButton.click();
}
};
};
只需将以上代码插入你需要的页面,并引用JS即可实现。